JAVA VARIABLE
- RAHUL KUMAR
- Jul 15, 2020
- 3 min read
Updated: Aug 1, 2020
Hello. We're going to start learning to execute code with the most basic statements in Java.
In Java, variable act as container used for storing data Values. Values assigned to variable can be changed during program execution.
Here, we have two variable declarations.
int X ;
int Y ;
One for an int called X and one for an int called Y.
But in this example, we have not provided any initial values. In some languages such as C, no default value is ever provided when you declare a variable. Means, you get undefined behavior if you use an uninitialized variable.
This is such a common problem that Java provides two solutions:
(1) an initial default value of zero is given to instance variables,
(2) an explicit error is given for using a local variable before initializing it.
Data Type Default Value (for fields)
byte 0
short 0
int 0
long 0L
float 0.0f
double 0.0d
char '\u0000'
String null
boolean false
Variable Declaration
To declare variable specify the data type before the variable name.
Syntax: datatype variableName ; or datatype variableName = datavalue ;
Example: String str = "World"; int number = 244; float decimal_num = 123.25f;
Now, let us look at another example which initializes the variables when they are declared.
int X = 4;
int Y = 6;
Here, the first statement declares X of datatype int and initializing the value 4 to it. In the next line
Y is declared of datatype int with initial value as 6.Initialization and declaration of variable in the same statement make your code clean and anyone reading your code knows exactly what you meant for the code to do.
uses of variables in code :
Above code declares X and initializes it to 4 and Y declares and initializes it to 6.
int Z = X + Y;
To perform this statement we must evaluate the expression on the right hand side.
X is 4 and Y is 6, so Z = 4 + 6 = 10.
int W = Y - X;
To perform this statement we must evaluate the expression on the right hand side.
X is 4 and Y is 6, so W = 6 - 4 = 2.
int X = 2;
int Y = X * 3;
int Z = Y / 2;
X = (Z + 2) % 2;
In the above code X having initial value 2 and on the next line Y = X * 3 = 2 * 3 = 6. In 3rd line
Z = Y / 2 = 6 / 2 = 3. Now the last expression is X = (Z + 2) % 2 = (3 + 2) % 2 = 5 % 2 = 1.
so, the value of X changes to 1. Therefore, if we print the value of X we will get 1 as output.
Great. Now, you know how to execute code with variable declarations and assignment statements.
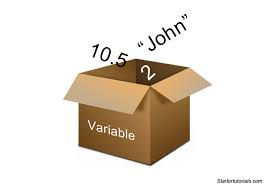
Comments